こんにちはコーヤです。
このページでは、SwiftUIのテキストのモディファイアをまとめてご紹介します。
以下のバージョンで動作確認しています。
- Xcode 13.4.1
- Swift 5.6.1
大きさの設定方法
定義済みの大きさを設定する
.font(文字の大きさ)
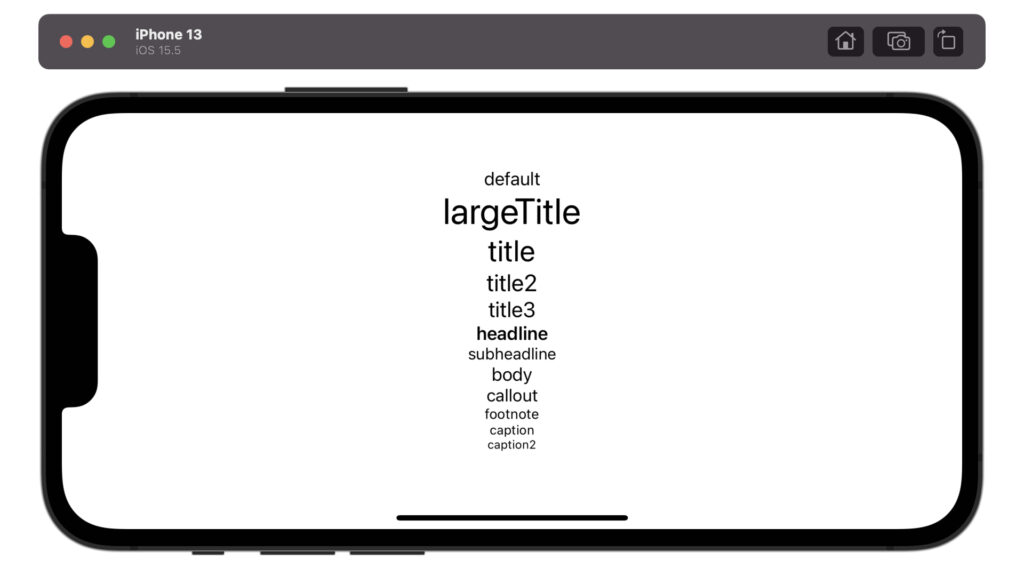
struct ContentView: View {
var body: some View {
VStack {
Group {
Text("default")
Text("largeTitle")
.font(.largeTitle)
Text("title")
.font(.title)
Text("title2")
.font(.title2)
Text("title3")
.font(.title3)
Text("headline")
.font(.headline)
}
Group {
Text("subheadline")
.font(.subheadline)
Text("body")
.font(.body)
Text("callout")
.font(.callout)
Text("footnote")
.font(.footnote)
Text("caption")
.font(.caption)
Text("caption2")
.font(.caption2)
}
}
}
}
指定の大きさを設定する
.font(.system(size: 文字の大きさ))
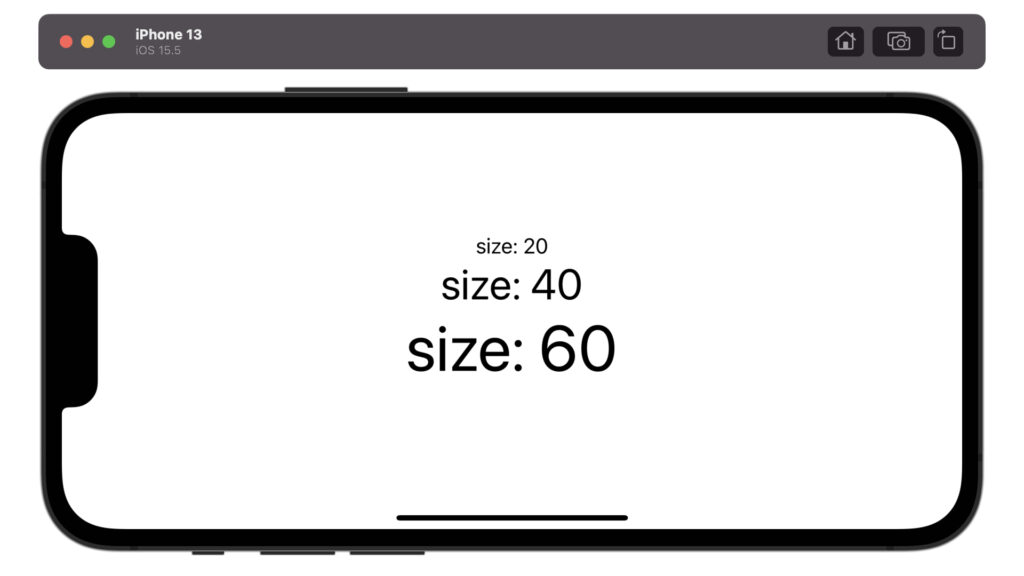
struct ContentView: View {
var body: some View {
VStack {
Text("size: 20")
.font(.system(size: 20))
Text("size: 40")
.font(.system(size: 40))
Text("size: 60")
.font(.system(size: 60))
}
}
}
太さの設定方法
.fontweight(文字の太さ)
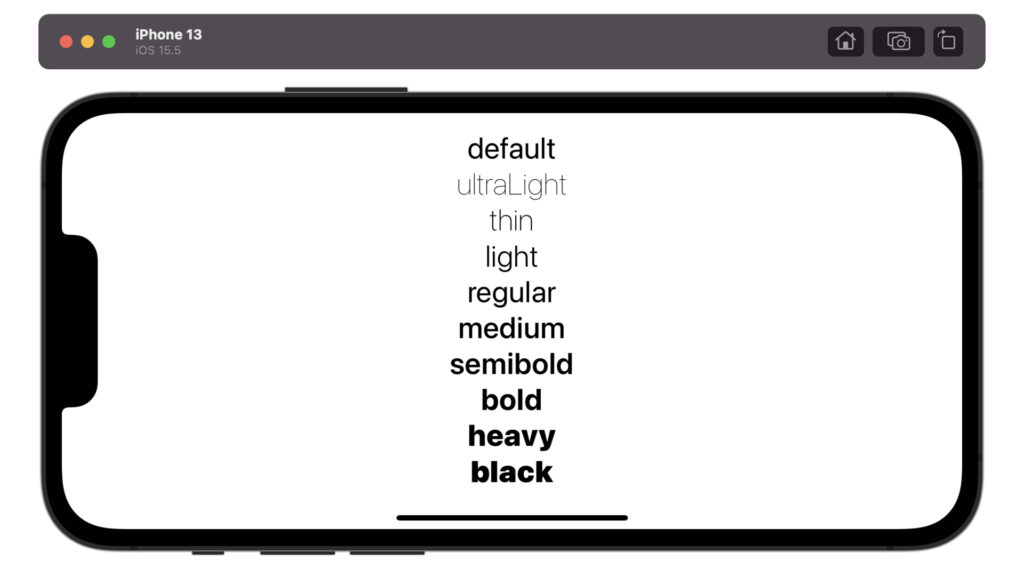
struct ContentView: View {
var body: some View {
VStack {
Text("default")
Text("ultraLight")
.fontWeight(.ultraLight)
Text("thin")
.fontWeight(.thin)
Text("light")
.fontWeight(.light)
Text("regular")
.fontWeight(.regular)
Text("medium")
.fontWeight(.medium)
Text("semibold")
.fontWeight(.semibold)
Text("bold")
.fontWeight(.bold)
Text("heavy")
.fontWeight(.heavy)
Text("black")
.fontWeight(.black)
}
.font(.title)
}
}
文字色の設定方法
.foregroundColor(文字の色)
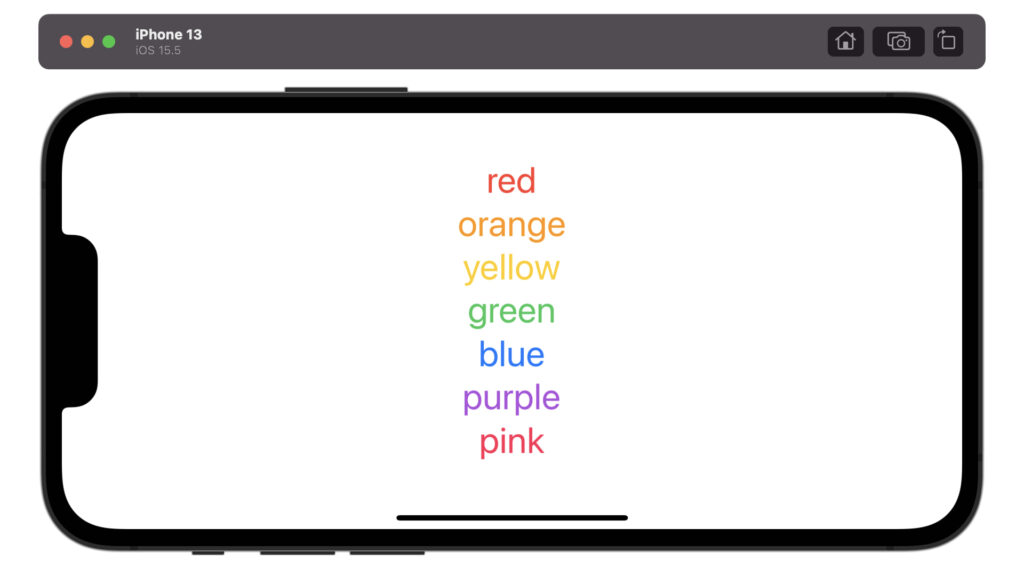
struct ContentView: View {
var body: some View {
VStack {
Text("red")
.foregroundColor(Color.red)
Text("orange")
.foregroundColor(Color.orange)
Text("yellow")
.foregroundColor(Color.yellow)
Text("green")
.foregroundColor(Color.green)
Text("blue")
.foregroundColor(Color.blue)
Text("purple")
.foregroundColor(Color.purple)
Text("pink")
.foregroundColor(Color.pink)
}
.font(.largeTitle)
}
}
背景色の設定方法
.background(背景の色)
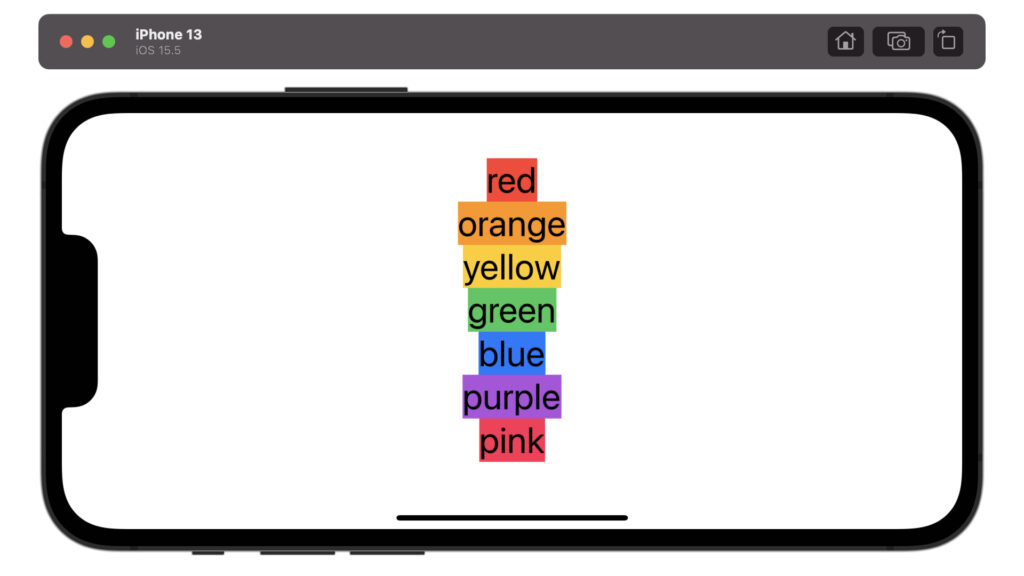
struct ContentView: View {
var body: some View {
VStack {
Text("red")
.background(.red)
Text("orange")
.background(.orange)
Text("yellow")
.background(.yellow)
Text("green")
.background(.green)
Text("blue")
.background(.blue)
Text("purple")
.background(.purple)
Text("pink")
.background(.pink)
}
.font(.largeTitle)
}
}
装飾の設定方法
.bold()
.italic()
.underline(color: 下線の色)
.strikethrough(color: 打ち消し線の色)
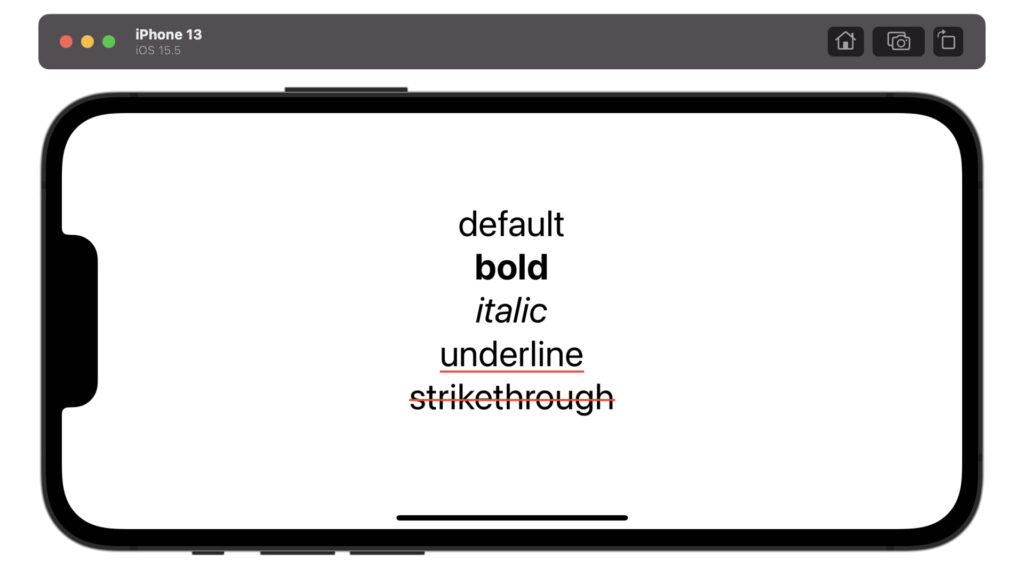
struct ContentView: View {
var body: some View {
VStack {
Text("default")
Text("bold")
.bold()
Text("italic")
.italic()
Text("underline")
.underline(color: .red)
Text("strikethrough")
.strikethrough(color: .red)
}
.font(.largeTitle)
}
}
文字間隔の設定方法
.kerning(文字間隔)
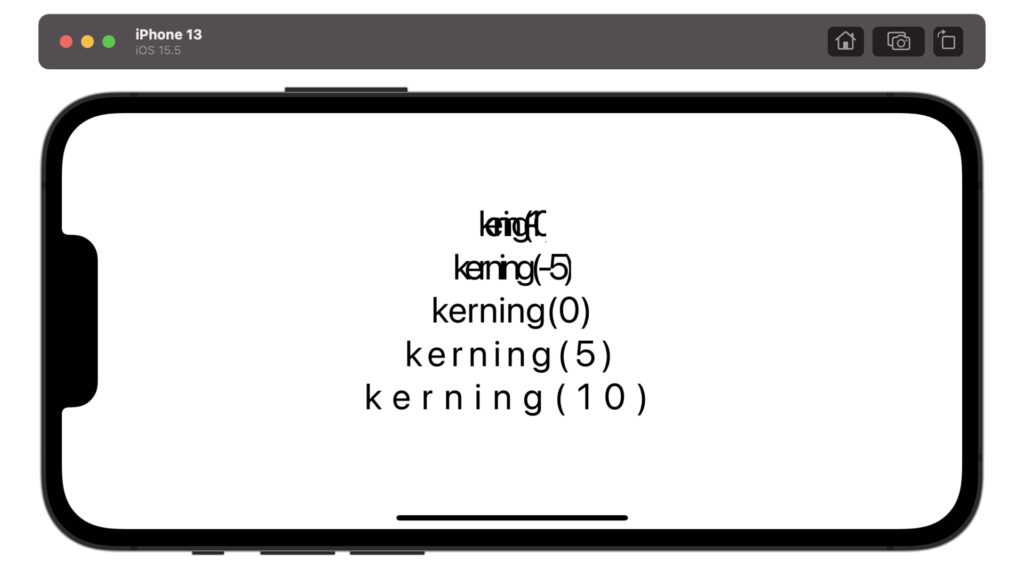
struct ContentView: View {
var body: some View {
VStack {
Text("kerning(-10)")
.kerning(-10)
Text("kerning(-5)")
.kerning(-5)
Text("kerning(0)")
.kerning(0)
Text("kerning(5)")
.kerning(5)
Text("kerning(10)")
.kerning(10)
}
.font(.largeTitle)
}
}
外枠の設定方法
.border(外枠の色 , width: 外枠の太さ)
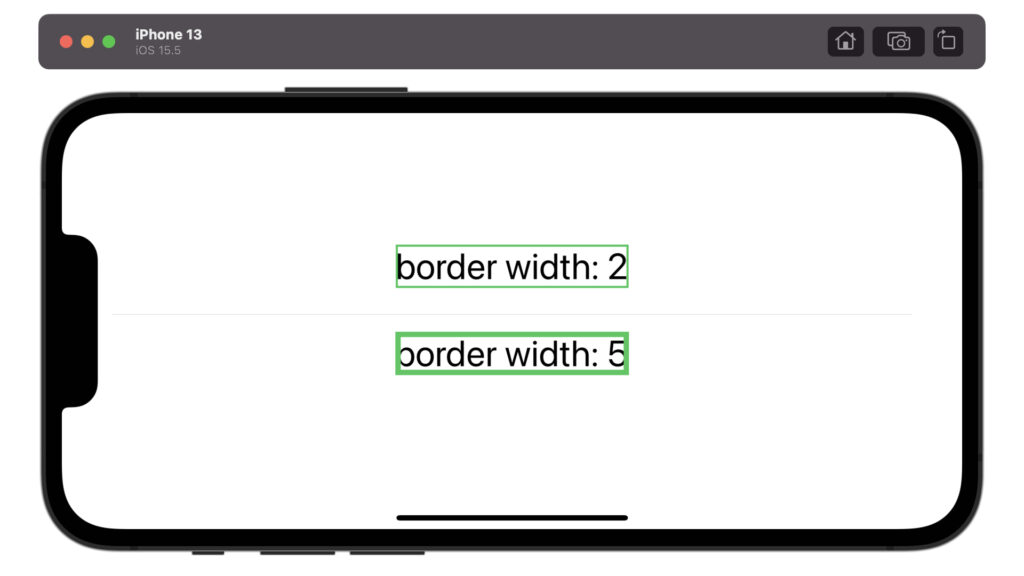
struct ContentView: View {
var body: some View {
VStack {
Text("border width: 2")
.border(Color.green , width: 2)
Divider()
Text("border width: 5")
.border(Color.green , width: 5)
}
.font(.largeTitle)
}
}
位置の設定方法
上下の位置を設定する
.baselineOffset(基準からのズレ)
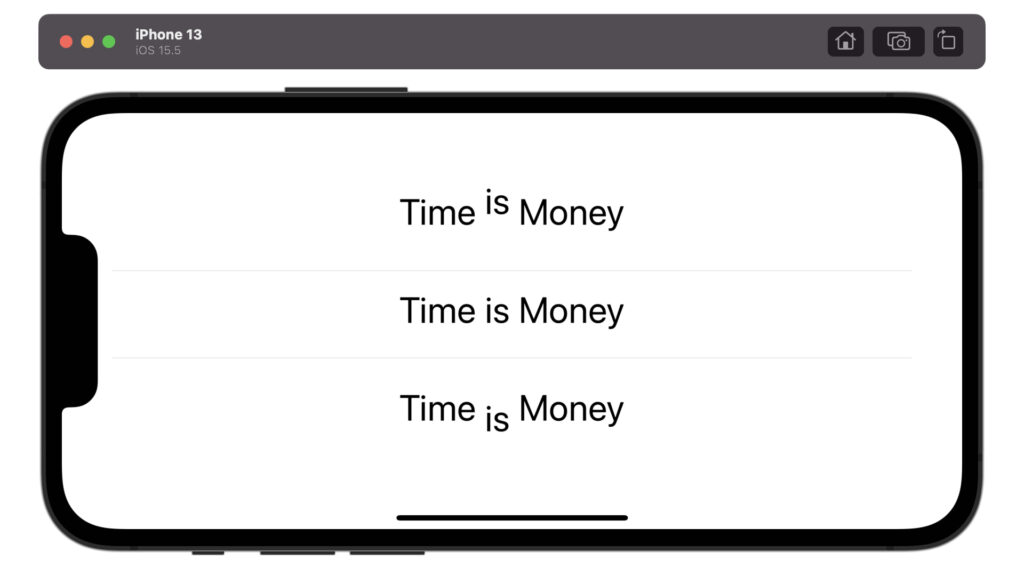
struct ContentView: View {
var body: some View {
VStack {
HStack {
Text("Time")
Text("is")
.baselineOffset(20)
Text("Money")
}
Divider()
HStack {
Text("Time")
Text("is")
.baselineOffset(0)
Text("Money")
}
Divider()
HStack {
Text("Time")
Text("is")
.baselineOffset(-20)
Text("Money")
}
}
.font(.largeTitle)
}
}
上下左右の位置を設定する
.offset(x: 横方向のズレ, y: 縦方向のズレ)
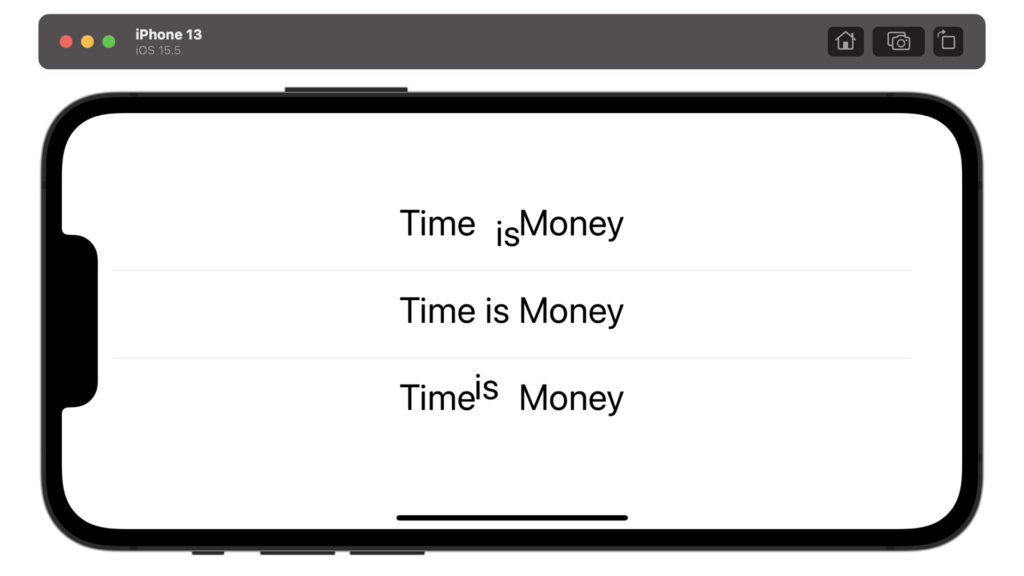
struct ContentView: View {
var body: some View {
VStack {
HStack {
Text("Time")
Text("is")
.offset(x: 10, y: 10)
Text("Money")
}
Divider()
HStack {
Text("Time")
Text("is")
.offset(x: 0, y: 0)
Text("Money")
}
Divider()
HStack {
Text("Time")
Text("is")
.offset(x: -10, y: -10)
Text("Money")
}
}
.font(.largeTitle)
}
}
フォントの設定方法
.font(.system(文字の大きさ , design: フォント))
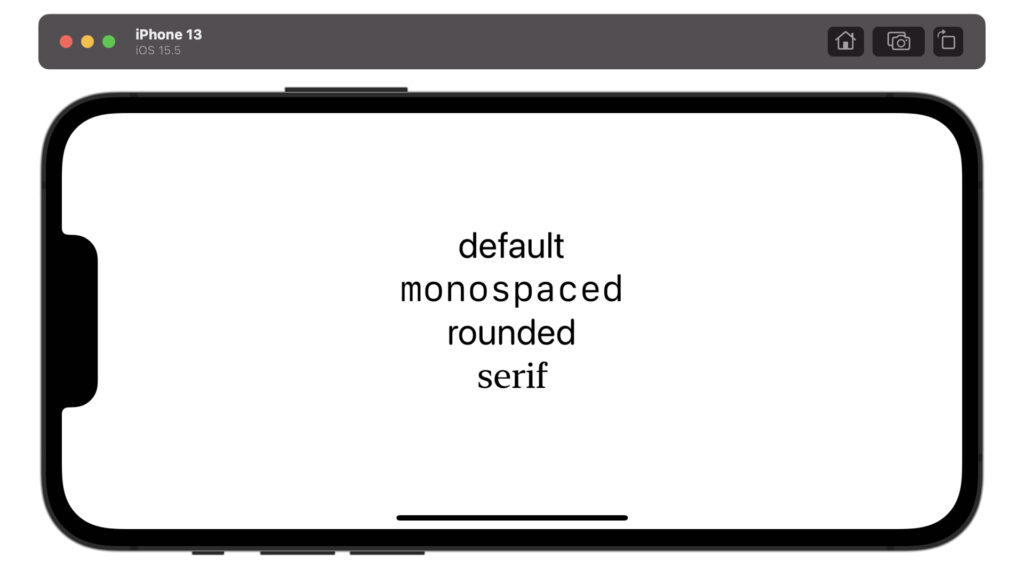
struct ContentView: View {
var body: some View {
VStack {
Text("default")
.font(.system(.largeTitle, design: .default))
Text("monospaced")
.font(.system(.largeTitle, design: .monospaced))
Text("rounded")
.font(.system(.largeTitle, design: .rounded))
Text("serif")
.font(.system(.largeTitle, design: .serif))
}
}
}
行間の設定方法
.lineSpacing(行間のスペース)
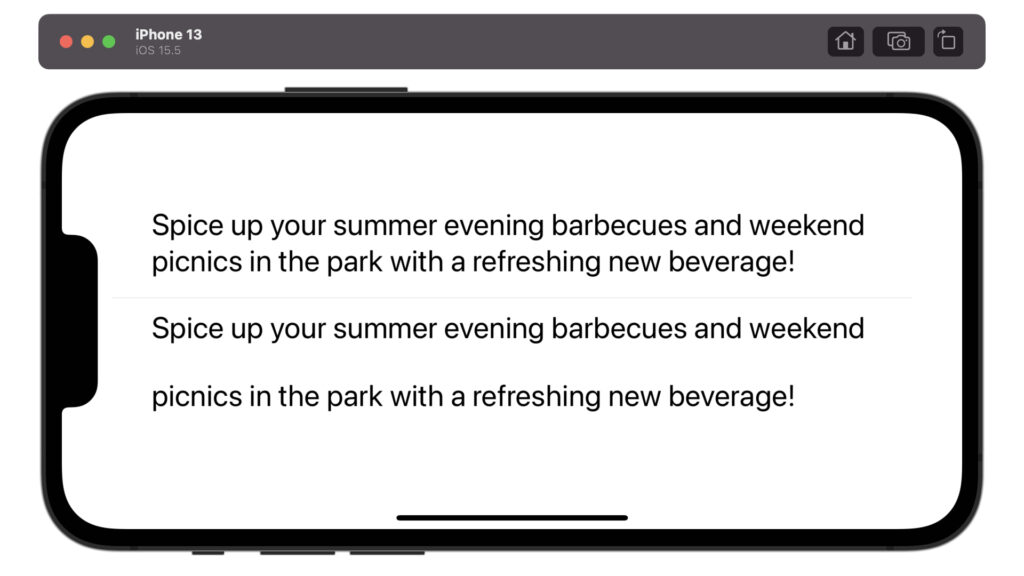
struct ContentView: View {
let sampleText: String = "Spice up your summer evening barbecues and weekend picnics in the park with a refreshing new beverage!"
var body: some View {
VStack {
Text(sampleText)
.lineSpacing(0)
Divider()
Text(sampleText)
.lineSpacing(30)
}
.font(.title)
}
}
位置揃えの設定方法
.multilineTextAlignment(位置揃えの基準)
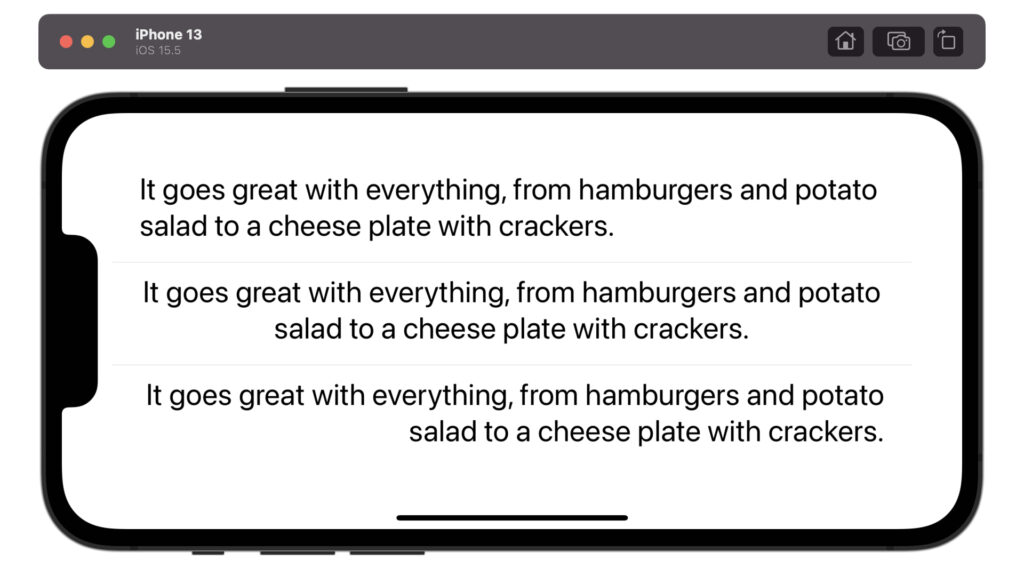
struct ContentView: View {
let sampleText: String = "It goes great with everything, from hamburgers and potato salad to a cheese plate with crackers."
var body: some View {
VStack {
Text(sampleText)
.multilineTextAlignment(.leading)
Divider()
Text(sampleText)
.multilineTextAlignment(.center)
Divider()
Text(sampleText)
.multilineTextAlignment(.trailing)
}
.font(.title)
}
}
表示範囲の設定方法
表示する範囲を設定する
.frame(width: 横の長さ, height: 縦の長さ)
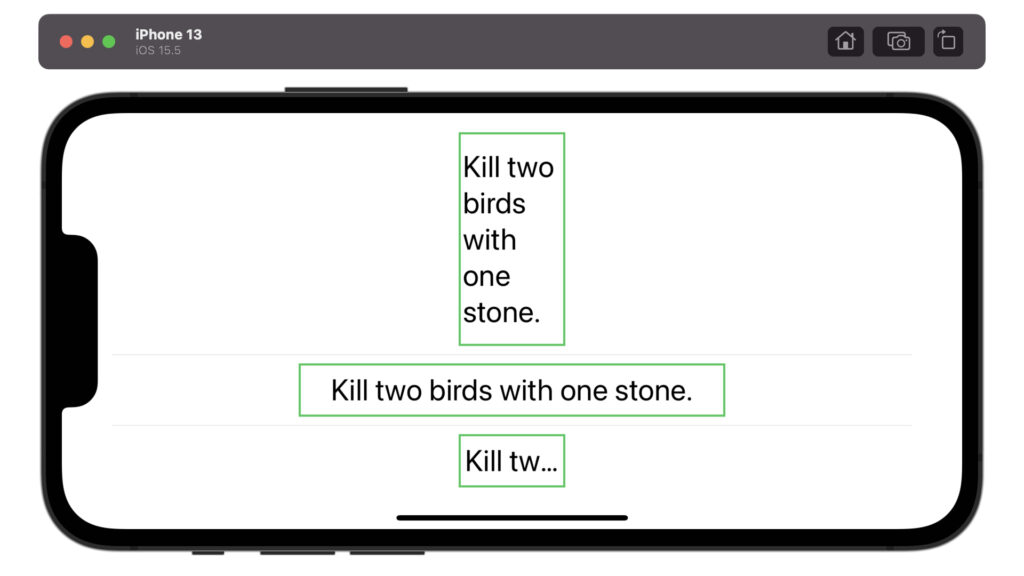
struct ContentView: View {
let sampleText: String = "Kill two birds with one stone."
var body: some View {
VStack {
Text(sampleText)
.frame(width: 100, height: 200)
.border(Color.green, width: 2)
Divider()
Text(sampleText)
.frame(width: 400, height: 50)
.border(Color.green, width: 2)
Divider()
Text(sampleText)
.frame(width: 100, height: 50)
.border(Color.green, width: 2)
}
.font(.title)
}
}
範囲内の位置揃えを設定する
.frame(width: 横の長さ, height: 縦の長さ, alignment: 表示する場所)
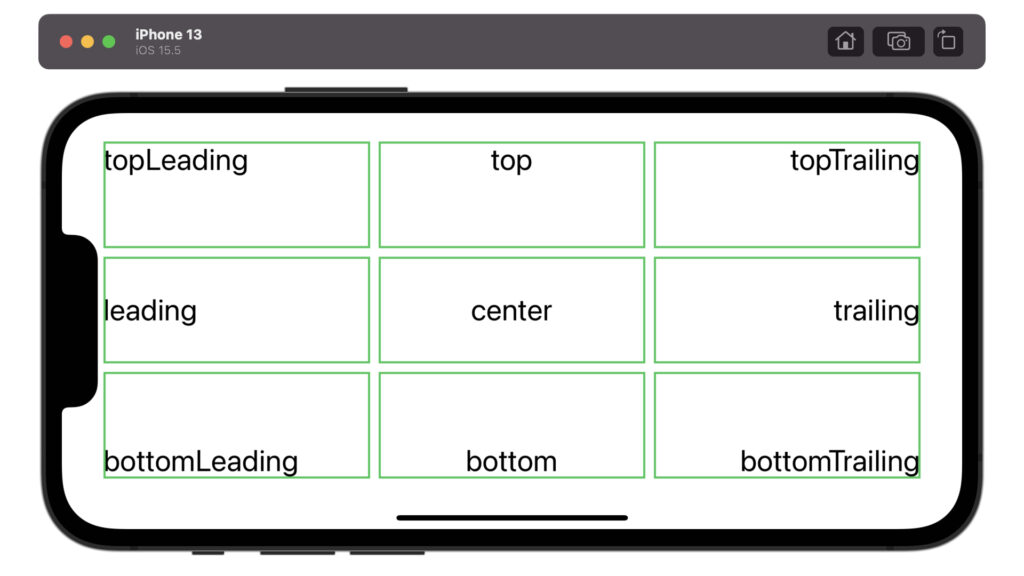
struct ContentView: View {
var body: some View {
VStack {
HStack {
Text("topLeading")
.frame(width: 250, height: 100, alignment: .topLeading)
.border(Color.green, width: 2)
Text("top")
.frame(width: 250, height: 100, alignment: .top)
.border(Color.green, width: 2)
Text("topTrailing")
.frame(width: 250, height: 100, alignment: .topTrailing)
.border(Color.green, width: 2)
}
HStack {
Text("leading")
.frame(width: 250, height: 100, alignment: .leading)
.border(Color.green, width: 2)
Text("center")
.frame(width: 250, height: 100, alignment: .center)
.border(Color.green, width: 2)
Text("trailing")
.frame(width: 250, height: 100, alignment: .trailing)
.border(Color.green, width: 2)
}
HStack {
Text("bottomLeading")
.frame(width: 250, height: 100, alignment: .bottomLeading)
.border(Color.green, width: 2)
Text("bottom")
.frame(width: 250, height: 100, alignment: .bottom)
.border(Color.green, width: 2)
Text("bottomTrailing")
.frame(width: 250, height: 100, alignment: .bottomTrailing)
.border(Color.green, width: 2)
}
}
.font(.title)
}
}
表示行数の設定方法
.lineLimit(行数の上限)
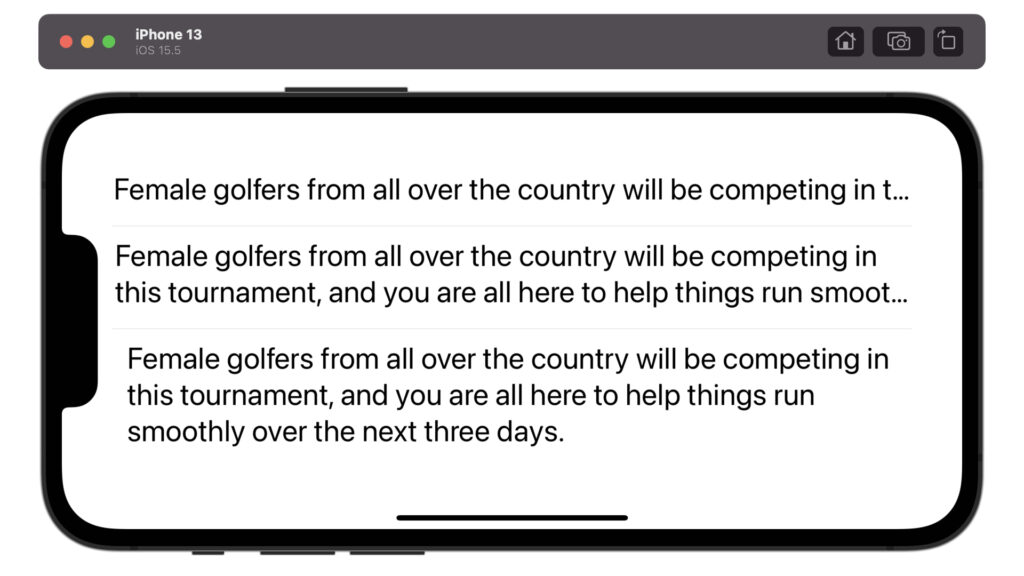
struct ContentView: View {
let sampleText: String = "Female golfers from all over the country will be competing in this tournament, and you are all here to help things run smoothly over the next three days."
var body: some View {
VStack {
Text(sampleText)
.lineLimit(1)
Divider()
Text(sampleText)
.lineLimit(2)
Divider()
Text(sampleText)
.lineLimit(3)
}
.font(.title)
}
}
省略位置の設定方法
.truncationMode(省略の位置)
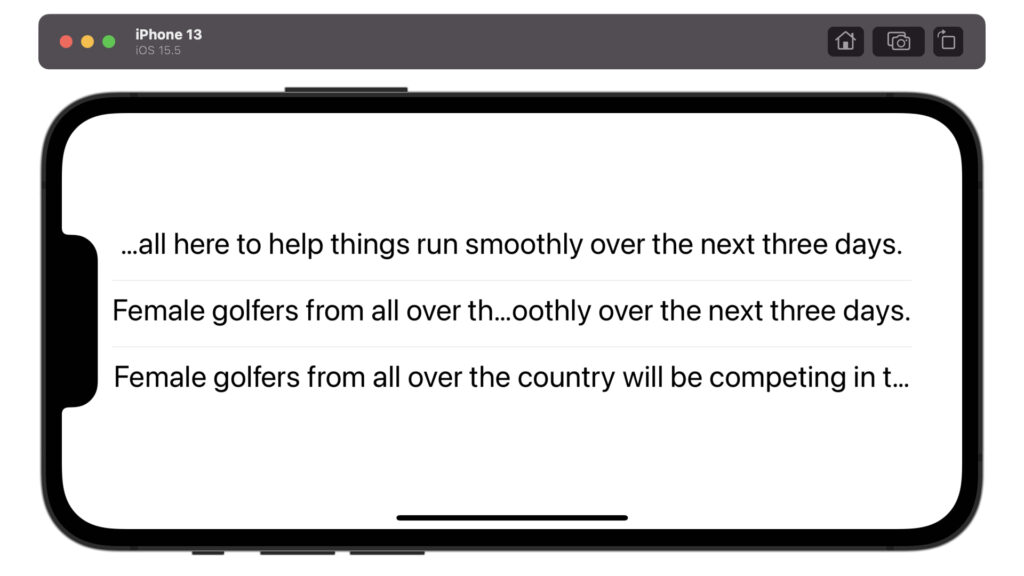
struct ContentView: View {
let sampleText: String = "Female golfers from all over the country will be competing in this tournament, and you are all here to help things run smoothly over the next three days."
var body: some View {
VStack {
Text(sampleText)
.truncationMode(.head)
Divider()
Text(sampleText)
.truncationMode(.middle)
Divider()
Text(sampleText)
.truncationMode(.tail)
}
.font(.title)
.lineLimit(1)
}
}
以上です。ご参考になれば幸いです。
コメント欄